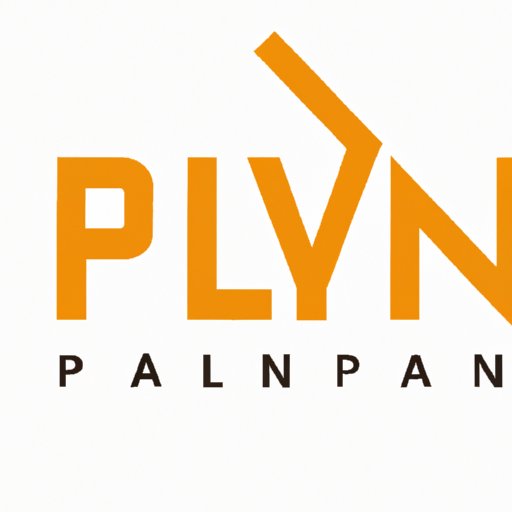
Introduction
String splitting is an essential task in Python programming that involves breaking a string into a list or multiple strings based on a specified delimiter. This operation is critical for data processing, text analysis, and string manipulation. As a Python developer, you’ll frequently encounter situations where you need to split a string into components to extract relevant information, perform calculations or comparisons, and convert data types.
In this article, we’ll explore various techniques for splitting strings in Python, ranging from basic string methods to advanced approaches using regular expressions and functional programming. We’ll provide code examples, compare and contrast different methods, and demonstrate how to apply them to real-world problems.
6 Simple and Easy Methods for Splitting Strings in Python
Python offers several built-in functions and methods for splitting strings, depending on your specific requirements and preferences. Here are six of the most common methods that you can use:
The split() Method
The split() method is one of the simplest and most intuitive ways of splitting strings in Python. It takes a specified delimiter as an argument and returns a list of substrings split at that delimiter. Here’s an example:
“`
s = “apple,banana,orange”
fruits = s.split(“,”)
print(fruits)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’]
“`
In this code, we define a string `s` containing a comma-separated list of three fruits. We then call the split() method on `s` and pass a comma as a delimiter. The method returns a list `fruits` containing the three fruits as separate strings.
The split() method is useful for simple string splitting tasks where the delimiter is a single character or a fixed pattern. However, it may not work well for more complex patterns or cases where the delimiter occurs in multiple places within the string. Additionally, the split() method can produce unexpected results if the delimiter appears at the beginning or end of the string.
The partition() Method
The partition() method is similar to the split() method, but it splits the string into three parts instead of two. Here’s an example:
“`
s = “apple:banana:orange”
first, delimiter, last = s.partition(“:”)
print(first, delimiter, last)
“`
Output:
“`
apple : banana : orange
“`
In this code, we define a string `s` containing a colon-separated list of three fruits. We then call the partition() method on `s` and pass a colon as a delimiter. The method returns three strings `first`, `delimiter`, and `last` representing the text before the delimiter, the delimiter itself, and the text after the delimiter.
The partition() method is useful when you need to split a string into two parts and preserve the delimiter. However, it may not work well for cases where the delimiter occurs multiple times within the string.
The rsplit() Method
The rsplit() method is similar to the split() method, but it splits the string from the right (i.e., starting at the end of the string) instead of the left. Here’s an example:
“`
s = “apple,banana,orange”
fruits = s.rsplit(“,”, 1)
print(fruits)
“`
Output:
“`
[‘apple,banana’, ‘orange’]
“`
In this code, we define a string `s` containing a comma-separated list of three fruits. We then call the rsplit() method on `s` and pass a comma as a delimiter and a limit of 1. The method returns a list `fruits` containing two strings, where the last occurrence of the delimiter is preserved and the other parts are split. The second argument specifies the maximum number of splits to perform, starting from the right.
The rsplit() method is useful when you only need to split a string once or when the delimiter may occur multiple times within the string but you want to preserve the last occurrence.
The splitlines() Method
The splitlines() method is a specialized method for splitting a string into separate lines. It takes no arguments and splits the string based on newline characters (`\n`), carriage return characters (`\r`), or a combination of both (`\r\n`). Here’s an example:
“`
s = “apple\nbanana\rorange\r\nmango”
lines = s.splitlines()
print(lines)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘mango’]
“`
In this code, we define a string `s` containing four fruits separated by different line endings. We then call the splitlines() method on `s`, and the method returns a list `lines` containing the four fruits as separate strings.
The splitlines() method is useful when you need to split a string into separate lines, such as when reading text files or processing multiline input.
The re.split() Method
The re (regular expression) module in Python provides advanced pattern matching and string manipulation capabilities, including string splitting. The re.split() method splits a string into substrings based on a regular expression pattern. Here’s an example:
“`
import re
s = “apple, banana, \norange”
words = re.split(“[,\s]+”, s)
print(words)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’]
“`
In this code, we import the re module, define a string `s` containing a comma-separated list of three fruits, and call the re.split() method on `s` with a regular expression pattern `”[,\s]+”`, which matches any combination of commas and whitespace characters. The method returns a list `words` containing the three fruits as separate strings.
The re.split() method is useful for cases where the delimiter is a complex pattern or when you need to split a string based on multiple delimiters. However, it may be slower than using built-in string methods for simple cases.
The str.split() Method with Unpacking
In Python 3.0 and later versions, a new way of using the split() method with unpacking was introduced. This method is similar to the partition() method in that it splits a string into three parts but uses unpacking to assign the parts to variables. Here’s an example:
“`
s = “apple:banana:orange”
first, *middle, last = s.split(“:”)
print(first, middle, last)
“`
Output:
“`
apple [‘banana’] orange
“`
In this code, we define a string `s` containing a colon-separated list of three fruits. We then call the split() method on `s` and pass a colon as a delimiter. The expressions `first`, `*middle`, and `last` use unpacking to assign the first part, any middle parts, and the last part to separate variables. The middle parts are collected into a list using the `*` syntax.
The str.split() method with unpacking is useful for cases where you need to split a string into multiple parts and handle each part separately.
Mastering String Splitting Techniques in Python
While the basic methods we’ve covered so far can handle most string splitting tasks, there are many cases where you may need more advanced techniques to handle complex patterns or optimize performance. Here are some advanced techniques for mastering string splitting in Python:
Using Regular Expressions
Regular expressions are a powerful tool for pattern matching and string manipulation in Python. They provide a concise and flexible way of specifying complex patterns that can handle a wide range of string splitting tasks. Here’s an example:
“`
import re
s = “apple:banana,orange.pear”
words = re.findall(r”\w+”, s)
print(words)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘pear’]
“`
In this code, we import the re module, define a string `s` containing a list of fruits separated by various punctuation characters, and call the re.findall() method with a regular expression pattern `”\w+”`, which matches any word character (i.e., letters, digits, and underscores). The method returns a list `words` containing the four fruits as separate strings.
Using regular expressions for string splitting allows you to match complex patterns that cannot be handled using simple delimiters or string methods. However, regular expressions can be tricky to write and optimize, and they may be slower than other methods for simple cases.
Using itertools
The itertools module in Python provides a range of powerful tools for iterable-based functions, including string manipulation. The itertools.chain() method, for example, can be used to concatenate multiple sequences or generators and produce a single iterable object that can then be split using a simple delimiter. Here’s an example:
“`
import itertools
s1 = “apple,banana”
s2 = “orange,pear”
fruits = “”.join(itertools.chain(s1, s2)).split(“,”)
print(fruits)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’, ‘pear’]
“`
In this code, we import the itertools module, define two strings `s1` and `s2` containing two fruits each separated by a comma, concatenate them using itertools.chain(), join the resulting iterable using “”.join(), and split the joined string using a comma delimiter. The method returns a list `fruits` containing the four fruits as separate strings.
Using itertools for string splitting allows you to handle multiple sequences or generators with ease and flexibility and produce complex iterables that can be used in other operations. However, itertools requires some programming skills and may be slightly slower than other methods for simple cases.
Using functools.reduce()
The functools module in Python provides advanced tools for functional programming, including the reduce() method, which allows you to apply a specified function to a sequence of elements cumulatively to obtain a single result. You can use this method to split a string based on a specified delimiter and accumulate the result into a list or other data structure. Here’s an example:
“`
import functools
s = “apple-banana-orange”
delimiter = “-”
words_list = functools.reduce(lambda lst, x: lst[:-1] + [lst[-1] + x] if x == delimiter else lst + [x], s, [”])
print(words_list)
“`
Output:
“`
[‘apple’, ‘banana’, ‘orange’]
“`
In this code, we import the functools module, define a string `s` containing a list of fruits separated by a hyphen, define a delimiter `-`, and use reduce() to apply a lambda function that splits the string into separate words based on the delimiter and accumulates the result into a list. The method returns a list `words_list` containing the three fruits as separate strings.
Using functools.reduce() for string splitting requires advanced programming skills and may be slower than other methods for simple cases. However, it allows you to perform complex transformations and accumulations on sequences of data in a functional and efficient way.
Splitting Strings in Python: A Comprehensive Guide with Examples
Now that we’ve covered basic and advanced techniques for string splitting in Python, let’s explore a comprehensive guide on how to split strings using different approaches, including real-world examples and best practices.
Step-by-Step Guide to Splitting Strings in Python
1. Determine the delimiter or pattern you want to use to split the string.
2. Choose the appropriate method for string splitting based on your specific requirements and preferences.
3. Apply the method to the string and store the result in a variable.
4. Handle any errors or exceptions that may occur during the string splitting process.
5. Validate the output to ensure it meets your expectations.
6. Further process the output as needed, such as converting it to a list, dictionary, or other data structure.
Real-World Examples and Use Cases
Here are some examples of how to split strings in Python in real-world use cases:
– Splitting log files: You can split a log file into separate entries based on a timestamp or other pattern using regular expressions or other advanced techniques.
– Parsing web pages: You can split a web page into different sections or components based on HTML tags or other markup using BeautifulSoup or other parsing libraries.